Useless candle : Différence entre versions
Ligne 43 : | Ligne 43 : | ||
==Etape 1 : mise en place de l'Arduino UNO == | ==Etape 1 : mise en place de l'Arduino UNO == | ||
+ | |||
+ | D'abord il faut télécharger le logiciel Arduino IDE (voir le lien) [https://www.arduino.cc/en/Main/Software là], l'installer et le lançer. | ||
+ | Ensuite choisir le type d'Arduino qu'on utilise de la boite Tools-->Board. Et puis il faut taper le code nécessaire au fonctionnement du projet | ||
[[Image:arduino.jpg|thumb|]] | [[Image:arduino.jpg|thumb|]] | ||
Ligne 49 : | Ligne 52 : | ||
==Etape 2 : Réalisation des scripts Arduino== | ==Etape 2 : Réalisation des scripts Arduino== | ||
+ | Le script Arduino est divisé en 2 fichiers (main.ino et tools.hpp) | ||
+ | |||
+ | ====Main==== | ||
+ | |||
+ | <code>/* Gasthon project | ||
+ | * | ||
+ | * main.ino | ||
+ | * | ||
+ | * members of the project : | ||
+ | * - YAGHI Anthony | ||
+ | * - SCHAAF Hugo | ||
+ | * - LANDURE Raphaël | ||
+ | * | ||
+ | * Author : SCHAAF Hugo | ||
+ | * | ||
+ | * IS3 - ENIB | ||
+ | * 01/2019 | ||
+ | */ | ||
+ | |||
+ | #include <OneWire.h> | ||
+ | #include <DallasTemperature.h> | ||
+ | #include <Servo.h> | ||
+ | |||
+ | #include "tools.hpp" | ||
+ | |||
+ | |||
+ | #define ONE_WIRE_BUS 3 // one wire sensors bus pin number | ||
+ | #define CANDLE_SENSOR 0 // index of the sensor (1st sensor so index 0) | ||
+ | #define BURNING_TEMP 26. // temperature threshold in deg celcius | ||
+ | |||
+ | // temperature sensor | ||
+ | OneWire oneWire{ONE_WIRE_BUS}; | ||
+ | DallasTemperature sensors{&oneWire}; | ||
+ | |||
+ | // ventilation management | ||
+ | tools::Ventilation ventilation{10, 11, 3000}; | ||
+ | |||
+ | //---------------------------------------------------------------------------// | ||
+ | void setup() | ||
+ | { | ||
+ | Serial.begin(9600); | ||
+ | sensors.begin(); | ||
+ | ventilation.begin(); | ||
+ | } | ||
+ | |||
+ | //---------------------------------------------------------------------------// | ||
+ | void loop() | ||
+ | { | ||
+ | // request temperature to the sensor | ||
+ | sensors.requestTemperatures(); | ||
+ | |||
+ | // get the temperature above the candle | ||
+ | float candleTemp = sensors.getTempCByIndex(CANDLE_SENSOR); | ||
+ | |||
+ | if(candleTemp != -127. ) | ||
+ | { | ||
+ | if(candleTemp >= BURNING_TEMP) ventilation.set(); | ||
+ | else ventilation.reset(); | ||
+ | tools::displayTemp(candleTemp); | ||
+ | } | ||
+ | |||
+ | ventilation.run(); | ||
+ | }</code> | ||
+ | |||
+ | ====Tools.hpp==== | ||
+ | |||
+ | <code>/* Gasthon project | ||
+ | * | ||
+ | * tools.hpp | ||
+ | * | ||
+ | * members of the project : | ||
+ | * - YAGHI Anthony | ||
+ | * - SCHAAF Hugo | ||
+ | * - LANDURE Raphaël | ||
+ | * | ||
+ | * Author : SCHAAF Hugo | ||
+ | * | ||
+ | * IS3 - ENIB | ||
+ | * 01/2019 | ||
+ | */ | ||
+ | |||
+ | #ifndef TOOLS_HPP | ||
+ | #define TOOLS_HPP | ||
+ | |||
+ | #include "Arduino.h" | ||
+ | #include <Servo.h> | ||
+ | |||
+ | // default delay to enter in emergency mode | ||
+ | #define DELAY 5000 // in ms | ||
+ | |||
+ | namespace tools | ||
+ | { | ||
+ | //---------------------------------------------------------------------------------------------// | ||
+ | |||
+ | struct Ventilation | ||
+ | { | ||
+ | // constructor | ||
+ | Ventilation(uint8_t pin0, uint8_t pin1, uint32_t wait_t= DELAY): | ||
+ | pin_balance{pin0}, pin_orient{pin1}, start_time{0}, wait_t{wait_t}, | ||
+ | emergency_count{false}, doRun{false} | ||
+ | { | ||
+ | }; | ||
+ | |||
+ | // run it once to initialize the ventilation system | ||
+ | void begin() | ||
+ | { | ||
+ | servo_balance.attach(pin_balance); | ||
+ | servo_orient.attach(pin_orient); | ||
+ | |||
+ | servo_balance.write(0); | ||
+ | servo_orient.write(0); | ||
+ | }; | ||
+ | |||
+ | uint8_t run() | ||
+ | { | ||
+ | // if no need to ventilate, exit | ||
+ | if(!doRun) return 0; | ||
+ | |||
+ | Serial.println("doRun"); | ||
+ | |||
+ | if(!emergency_count) | ||
+ | { | ||
+ | emergency_count = true; | ||
+ | start_time = millis(); | ||
+ | } | ||
+ | |||
+ | if(millis() - start_time > wait_t) | ||
+ | { | ||
+ | Serial.println("emergency"); | ||
+ | servo_orient.write(90); | ||
+ | } | ||
+ | |||
+ | servo_balance.write(20); | ||
+ | delay(250); | ||
+ | servo_balance.write(0); | ||
+ | delay(250); | ||
+ | |||
+ | return 1; | ||
+ | }; | ||
+ | |||
+ | void set() | ||
+ | { | ||
+ | if(!doRun) | ||
+ | { | ||
+ | Serial.println("set"); | ||
+ | doRun = true; | ||
+ | } | ||
+ | }; | ||
+ | |||
+ | // reset to initial position | ||
+ | void reset() | ||
+ | { | ||
+ | |||
+ | if(doRun) | ||
+ | { | ||
+ | Serial.println("reset"); | ||
+ | servo_balance.write(0); | ||
+ | servo_orient.write(0); | ||
+ | emergency_count = false; | ||
+ | doRun = false; | ||
+ | } | ||
+ | }; | ||
+ | |||
+ | // motorisation members | ||
+ | Servo servo_balance, servo_orient; | ||
+ | uint8_t pin_balance, pin_orient; | ||
+ | // timer members | ||
+ | int32_t start_time; | ||
+ | uint32_t wait_t; | ||
+ | // flags | ||
+ | bool emergency_count, doRun; | ||
+ | }; | ||
+ | |||
+ | //---------------------------------------------------------------------------------------------// | ||
+ | |||
+ | inline | ||
+ | void displayTemp(float temp) | ||
+ | { | ||
+ | static float prev_temp = temp+1.; | ||
+ | if(temp > prev_temp + 0.3 || temp < prev_temp - 0.3) | ||
+ | { | ||
+ | prev_temp = temp; | ||
+ | Serial.println("candle temperature : "+String(temp)+" °C"); | ||
+ | } | ||
+ | }; | ||
+ | |||
+ | }// namespace tools | ||
+ | |||
+ | #endif</code> | ||
+ | ***Pour télécharger les fichiers :) | ||
+ | [[Fichier:main.ino]] ~ [[Fichier:tools.hpp]] | ||
==Etape 3 : Création de la boite== | ==Etape 3 : Création de la boite== |
Version du 21 janvier 2019 à 14:47
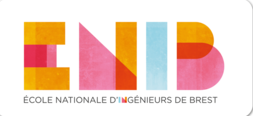
Réalisé par le FEU AU Q
Sommaire
Description du projet
Ce projet permet d'aborder plusieurs notions :
- Utilisation d'un arduino
- Programmation arduino
- Gestion d'un servomoteur
- Gestion des capteurs
- Design de la boite (CATIA)
Équipe du projet
*YAGHI Anthony *SCHAAF Hugo *LANDURE Raphael
Liste du Materiel
Materiel de base
- Arduino UNO
- thermomètre 1wire DS18B20
- Breadboard
- LED RGB
- Câbles
- Parois de la bougie : bois découpé avec découpeuse laser
- 2 Servomoteurs
- Elastiques
- 1 Cuillère plastique mcdo mcflurry :)
- Bougie
Materiel rajouté
Tutoriel
Etape 1 : mise en place de l'Arduino UNO
D'abord il faut télécharger le logiciel Arduino IDE (voir le lien) là, l'installer et le lançer. Ensuite choisir le type d'Arduino qu'on utilise de la boite Tools-->Board. Et puis il faut taper le code nécessaire au fonctionnement du projet
Etape 2 : Réalisation des scripts Arduino
Le script Arduino est divisé en 2 fichiers (main.ino et tools.hpp)
Main
/* Gasthon project
* * main.ino * * members of the project : * - YAGHI Anthony * - SCHAAF Hugo * - LANDURE Raphaël * * Author : SCHAAF Hugo * * IS3 - ENIB * 01/2019 */
- include <OneWire.h>
- include <DallasTemperature.h>
- include <Servo.h>
- include "tools.hpp"
- define ONE_WIRE_BUS 3 // one wire sensors bus pin number
- define CANDLE_SENSOR 0 // index of the sensor (1st sensor so index 0)
- define BURNING_TEMP 26. // temperature threshold in deg celcius
// temperature sensor OneWire oneWire{ONE_WIRE_BUS}; DallasTemperature sensors{&oneWire};
// ventilation management tools::Ventilation ventilation{10, 11, 3000};
//---------------------------------------------------------------------------// void setup() { Serial.begin(9600); sensors.begin(); ventilation.begin(); }
//---------------------------------------------------------------------------// void loop() { // request temperature to the sensor sensors.requestTemperatures();
// get the temperature above the candle float candleTemp = sensors.getTempCByIndex(CANDLE_SENSOR);
if(candleTemp != -127. ) { if(candleTemp >= BURNING_TEMP) ventilation.set(); else ventilation.reset(); tools::displayTemp(candleTemp); }
ventilation.run(); }
Tools.hpp
/* Gasthon project
* * tools.hpp * * members of the project : * - YAGHI Anthony * - SCHAAF Hugo * - LANDURE Raphaël * * Author : SCHAAF Hugo * * IS3 - ENIB * 01/2019 */
- ifndef TOOLS_HPP
- define TOOLS_HPP
- include "Arduino.h"
- include <Servo.h>
// default delay to enter in emergency mode
- define DELAY 5000 // in ms
namespace tools { //---------------------------------------------------------------------------------------------//
struct Ventilation { // constructor Ventilation(uint8_t pin0, uint8_t pin1, uint32_t wait_t= DELAY): pin_balance{pin0}, pin_orient{pin1}, start_time{0}, wait_t{wait_t}, emergency_count{false}, doRun{false} { };
// run it once to initialize the ventilation system void begin() { servo_balance.attach(pin_balance); servo_orient.attach(pin_orient);
servo_balance.write(0); servo_orient.write(0); };
uint8_t run() { // if no need to ventilate, exit if(!doRun) return 0;
Serial.println("doRun");
if(!emergency_count) { emergency_count = true; start_time = millis(); }
if(millis() - start_time > wait_t) { Serial.println("emergency"); servo_orient.write(90); }
servo_balance.write(20); delay(250); servo_balance.write(0); delay(250);
return 1; };
void set() { if(!doRun) { Serial.println("set"); doRun = true; } };
// reset to initial position void reset() {
if(doRun) { Serial.println("reset"); servo_balance.write(0); servo_orient.write(0); emergency_count = false; doRun = false; } };
// motorisation members Servo servo_balance, servo_orient; uint8_t pin_balance, pin_orient; // timer members int32_t start_time; uint32_t wait_t; // flags bool emergency_count, doRun; };
//---------------------------------------------------------------------------------------------//
inline void displayTemp(float temp) { static float prev_temp = temp+1.; if(temp > prev_temp + 0.3 || temp < prev_temp - 0.3) { prev_temp = temp; Serial.println("candle temperature : "+String(temp)+" °C"); } };
}// namespace tools
- endif
- Pour télécharger les fichiers :)
Fichier:Main.ino ~ Fichier:Tools.hpp